Open the site in SharePoint Desinger
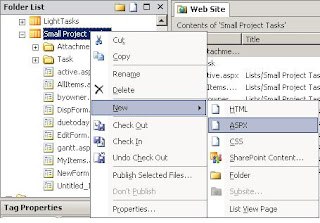
The page will open. Select Insert on the command line -> SharePoint Controls -> Custom List Form
A popup form will display the lists in the web, select the list you want, the content type, the type of form and check Show standard toolbar. Click ok.
Rename the page if you would like, untitled.aspx isn't appealing to me.
<SharePoint:CssLink runat="server"/>
<SharePoint:Theme runat="server"/>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$("input[id*='savebutton']").removeAttr('onclick');
$("input[id*='savebutton']").click(function(){
if (!PreSaveItem())
{ return false; }
var ctlName = $(this).attr('name');
setTimeout(function(){
WebForm_DoPostBackWithOptions(new WebForm_PostBackOptions(ctlName, "", true, "", "", false, true));
setTimeout(function(){parent.$.modal.close();},1);
},1);
});
$("input[id*='gobackbutton']").click(function(){
parent.$.modal.close();
});
});
</script>
<SharePoint:Theme runat="server"/>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$("input[id*='savebutton']").removeAttr('onclick');
$("input[id*='savebutton']").click(function(){
if (!PreSaveItem())
{ return false; }
var ctlName = $(this).attr('name');
setTimeout(function(){
WebForm_DoPostBackWithOptions(new WebForm_PostBackOptions(ctlName, "", true, "", "", false, true));
setTimeout(function(){parent.$.modal.close();},1);
},1);
});
$("input[id*='gobackbutton']").click(function(){
parent.$.modal.close();
});
});
</script>
Finally Add a Title for the new page
Select the first area of the page, the outlined box or the highlighted <td> tag. Replace that tag with this one.
<td width="99%" nowrap=""><SharePoint:ListProperty Property="Title" runat="server"/></td>
That's it your done! This page is ready to be used in a lightbox.